Contenido#
%%capture --no-display
import matplotlib.pyplot as plt
import numpy as np
with plt.xkcd():
x = np.linspace(0, 10, 100)
y = np.exp(x)
plt.plot(x,y)
plt.title("Cosas que puedes hacer en Python cuando aprendes.")
plt.xlabel("Días")
plt.ylabel("Número de cosas")
plt.show()
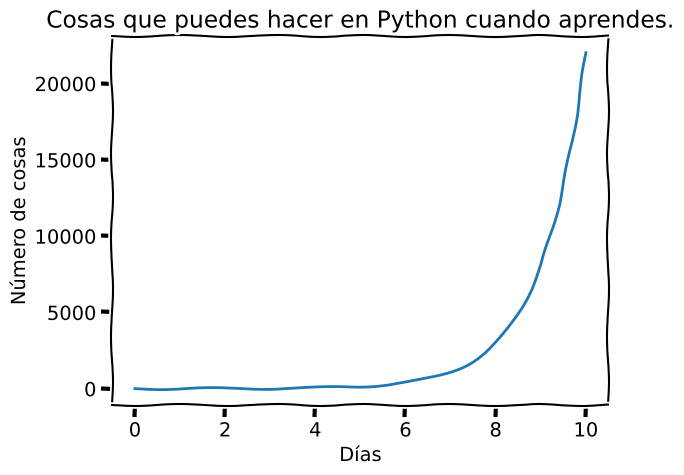
Los siguientes contenidos serán presentados en una sesión de videoconferencia. Puedes encontrar en este enlace las slides usadas como soporte.
Gestión de ficheros#
Veamos ahora como puedo abrir un fichero para escribir información en disco.
mi_fichero = open('fichero_de_prueba.txt', 'w') # 'w' especifica que el fichero permite la escritura
type(mi_fichero)
_io.TextIOWrapper
Si estás ejecutando este notebook de manera local con tu jupyter lab, verás que en el navegador de archivos de tu izquierda acaba de aparecer el nuevo fichero.
mi_fichero.write('hola!\n') # \n sirve como finalizar linea
6
mi_fichero.write('esta es la segunda linea\n')
25
mi_fichero
es un objeto definido por Python para entrada y salida de datos. Tiene métodos como write()
cuya función es operar con el objeto. En este caso write()
escribe en el fichero y devuelve como salida la cantidad de bytes escritos. Otro método que necesitarás es close()
mi_fichero.close()
print('Está el fichero cerrado?')
print(mi_fichero.closed) # `close` es un atributo que registra True o False según esté cerrado o abierto.
Está el fichero cerrado?
True
Ahora abriremos el fichero y lo leeremos. Pero antes, vamos a borrar mi fichero para hacer el caso más realista, lo habitual es que leamos un fichero pre-existente.
del(mi_fichero)
# Protegemos el fichero contra escritura declarándolo de lectura con 'r'
mi_fichero = open('fichero_de_prueba.txt', 'r')
contenido = mi_fichero.read()
mi_fichero.close()
print(contenido)
hola!
esta es la segunda linea
del(mi_fichero)
mi_fichero = open('fichero_de_prueba.txt', 'r')
primera_linea = mi_fichero.readline()
segunda_linea = mi_fichero.readline()
mi_fichero.close()
del(mi_fichero)
print(segunda_linea)
esta es la segunda linea
Ya podemos antes de concluir esta sección borrar el fichero. Esto lo puedes hacer de dos maneras:
Puedes acudir al navegador de archivos de Jupyter y con el botón derecho sobre “fichero_de_prueba.txt” seleccionar “Delete”.
Puedes importar la librería de python
os
y usar uno de sus métodos cuya función es borrar ficheros del disco duro. Veamos este ejemplo en las siguientes celdas.
import os
dir(os)
['CLD_CONTINUED',
'CLD_DUMPED',
'CLD_EXITED',
'CLD_KILLED',
'CLD_STOPPED',
'CLD_TRAPPED',
'DirEntry',
'EFD_CLOEXEC',
'EFD_NONBLOCK',
'EFD_SEMAPHORE',
'EX_CANTCREAT',
'EX_CONFIG',
'EX_DATAERR',
'EX_IOERR',
'EX_NOHOST',
'EX_NOINPUT',
'EX_NOPERM',
'EX_NOUSER',
'EX_OK',
'EX_OSERR',
'EX_OSFILE',
'EX_PROTOCOL',
'EX_SOFTWARE',
'EX_TEMPFAIL',
'EX_UNAVAILABLE',
'EX_USAGE',
'F_LOCK',
'F_OK',
'F_TEST',
'F_TLOCK',
'F_ULOCK',
'GenericAlias',
'Mapping',
'MutableMapping',
'NGROUPS_MAX',
'O_ACCMODE',
'O_APPEND',
'O_ASYNC',
'O_CLOEXEC',
'O_CREAT',
'O_DIRECT',
'O_DIRECTORY',
'O_DSYNC',
'O_EXCL',
'O_FSYNC',
'O_LARGEFILE',
'O_NDELAY',
'O_NOATIME',
'O_NOCTTY',
'O_NOFOLLOW',
'O_NONBLOCK',
'O_RDONLY',
'O_RDWR',
'O_RSYNC',
'O_SYNC',
'O_TRUNC',
'O_WRONLY',
'POSIX_FADV_DONTNEED',
'POSIX_FADV_NOREUSE',
'POSIX_FADV_NORMAL',
'POSIX_FADV_RANDOM',
'POSIX_FADV_SEQUENTIAL',
'POSIX_FADV_WILLNEED',
'POSIX_SPAWN_CLOSE',
'POSIX_SPAWN_DUP2',
'POSIX_SPAWN_OPEN',
'PRIO_PGRP',
'PRIO_PROCESS',
'PRIO_USER',
'P_ALL',
'P_NOWAIT',
'P_NOWAITO',
'P_PGID',
'P_PID',
'P_WAIT',
'PathLike',
'RTLD_DEEPBIND',
'RTLD_GLOBAL',
'RTLD_LAZY',
'RTLD_LOCAL',
'RTLD_NODELETE',
'RTLD_NOLOAD',
'RTLD_NOW',
'R_OK',
'SCHED_BATCH',
'SCHED_FIFO',
'SCHED_IDLE',
'SCHED_OTHER',
'SCHED_RESET_ON_FORK',
'SCHED_RR',
'SEEK_CUR',
'SEEK_END',
'SEEK_SET',
'SPLICE_F_MORE',
'SPLICE_F_MOVE',
'SPLICE_F_NONBLOCK',
'ST_APPEND',
'ST_MANDLOCK',
'ST_NOATIME',
'ST_NODEV',
'ST_NODIRATIME',
'ST_NOEXEC',
'ST_NOSUID',
'ST_RDONLY',
'ST_RELATIME',
'ST_SYNCHRONOUS',
'ST_WRITE',
'TMP_MAX',
'WCONTINUED',
'WCOREDUMP',
'WEXITED',
'WEXITSTATUS',
'WIFCONTINUED',
'WIFEXITED',
'WIFSIGNALED',
'WIFSTOPPED',
'WNOHANG',
'WNOWAIT',
'WSTOPPED',
'WSTOPSIG',
'WTERMSIG',
'WUNTRACED',
'W_OK',
'XATTR_CREATE',
'XATTR_REPLACE',
'XATTR_SIZE_MAX',
'X_OK',
'_Environ',
'__all__',
'__builtins__',
'__cached__',
'__doc__',
'__file__',
'__loader__',
'__name__',
'__package__',
'__spec__',
'_check_methods',
'_execvpe',
'_exists',
'_exit',
'_fspath',
'_fwalk',
'_get_exports_list',
'_spawnvef',
'_walk',
'_wrap_close',
'abc',
'abort',
'access',
'altsep',
'chdir',
'chmod',
'chown',
'chroot',
'close',
'closerange',
'confstr',
'confstr_names',
'cpu_count',
'ctermid',
'curdir',
'defpath',
'device_encoding',
'devnull',
'dup',
'dup2',
'environ',
'environb',
'error',
'eventfd',
'eventfd_read',
'eventfd_write',
'execl',
'execle',
'execlp',
'execlpe',
'execv',
'execve',
'execvp',
'execvpe',
'extsep',
'fchdir',
'fchmod',
'fchown',
'fdatasync',
'fdopen',
'fork',
'forkpty',
'fpathconf',
'fsdecode',
'fsencode',
'fspath',
'fstat',
'fstatvfs',
'fsync',
'ftruncate',
'fwalk',
'get_blocking',
'get_exec_path',
'get_inheritable',
'get_terminal_size',
'getcwd',
'getcwdb',
'getegid',
'getenv',
'getenvb',
'geteuid',
'getgid',
'getgrouplist',
'getgroups',
'getloadavg',
'getlogin',
'getpgid',
'getpgrp',
'getpid',
'getppid',
'getpriority',
'getresgid',
'getresuid',
'getsid',
'getuid',
'getxattr',
'initgroups',
'isatty',
'kill',
'killpg',
'lchown',
'linesep',
'link',
'listdir',
'listxattr',
'lockf',
'lseek',
'lstat',
'major',
'makedev',
'makedirs',
'minor',
'mkdir',
'mkfifo',
'mknod',
'name',
'nice',
'open',
'openpty',
'pardir',
'path',
'pathconf',
'pathconf_names',
'pathsep',
'pipe',
'pipe2',
'popen',
'posix_fadvise',
'posix_fallocate',
'posix_spawn',
'posix_spawnp',
'pread',
'preadv',
'putenv',
'pwrite',
'pwritev',
'read',
'readlink',
'readv',
'register_at_fork',
'remove',
'removedirs',
'removexattr',
'rename',
'renames',
'replace',
'rmdir',
'scandir',
'sched_get_priority_max',
'sched_get_priority_min',
'sched_getaffinity',
'sched_getparam',
'sched_getscheduler',
'sched_param',
'sched_rr_get_interval',
'sched_setaffinity',
'sched_setparam',
'sched_setscheduler',
'sched_yield',
'sendfile',
'sep',
'set_blocking',
'set_inheritable',
'setegid',
'seteuid',
'setgid',
'setgroups',
'setpgid',
'setpgrp',
'setpriority',
'setregid',
'setresgid',
'setresuid',
'setreuid',
'setsid',
'setuid',
'setxattr',
'spawnl',
'spawnle',
'spawnlp',
'spawnlpe',
'spawnv',
'spawnve',
'spawnvp',
'spawnvpe',
'splice',
'st',
'stat',
'stat_result',
'statvfs',
'statvfs_result',
'strerror',
'supports_bytes_environ',
'supports_dir_fd',
'supports_effective_ids',
'supports_fd',
'supports_follow_symlinks',
'symlink',
'sync',
'sys',
'sysconf',
'sysconf_names',
'system',
'tcgetpgrp',
'tcsetpgrp',
'terminal_size',
'times',
'times_result',
'truncate',
'ttyname',
'umask',
'uname',
'uname_result',
'unlink',
'unsetenv',
'urandom',
'utime',
'wait',
'wait3',
'wait4',
'waitid',
'waitid_result',
'waitpid',
'waitstatus_to_exitcode',
'walk',
'write',
'writev']
help(os.remove)
Help on built-in function remove in module posix:
remove(path, *, dir_fd=None)
Remove a file (same as unlink()).
If dir_fd is not None, it should be a file descriptor open to a directory,
and path should be relative; path will then be relative to that directory.
dir_fd may not be implemented on your platform.
If it is unavailable, using it will raise a NotImplementedError.
os.remove('fichero_de_prueba.txt')
Si has optado por borrar el fichero haciendo uso de la librería os
, puedes comprobar que este desapareció del navegador de archivos de Jupyter Lab que se encuentra a la izquierda de este notebook.
Numpy#
Para introducir Numpy, te sugerimos que sigas el contenido de este jupyter notebook.
Matplotlib#
Para introducir Matplotlib, te sugerimos que sigas el contenido de este jupyter notebook.